關(guān)聯(lián)數(shù)組實際上是一種查找表,內(nèi)存空間直到被使用時才會分配,每個數(shù)據(jù)項都會有一個特定的“鍵(索引)”,索引的類型不局限于整型。
相對于一般的數(shù)組,關(guān)聯(lián)數(shù)組的內(nèi)存空間利用會更加充分,因為它是稀疏的,無需一開始就分配很大的內(nèi)存空間。
當(dāng)變量集合的大小未知或數(shù)據(jù)空間稀疏時,關(guān)聯(lián)數(shù)組是比動態(tài)數(shù)組更好的選擇。例如只需要使用到地址0和地址FF,關(guān)聯(lián)數(shù)組可以只分配2個地址空間,而動態(tài)數(shù)組則需要分配256個地址空間。
打個比方,如果動態(tài)數(shù)組時內(nèi)存,那么關(guān)聯(lián)數(shù)組更像是一個具有“tag”的cache。
關(guān)聯(lián)數(shù)組也是一種unpacked數(shù)組。
data_type array_id [index_type];
其中data_type是數(shù)組元素的數(shù)據(jù)類型。array_id是數(shù)組的名稱。index_type是索引(或鍵)的數(shù)據(jù)類型。各種數(shù)據(jù)類型的索引示例如下:
//wildcard index. Can be indexed by any integral type int myArray[ * ]; //Array that stores 'bit [31:0]' at string type index. bit [31:0] myArray[ string ]; //Array that stores 'string' at string type index. string myArray [ string ]; // Array that stores 'int' at Class type index int myArray [ class ]; //Array that stores 'logic' type at integer type index logic myArray[ integer ]; typedef bit signed [7:0] mByte; int myArray [mByte]; //'bit signed' index
比較特別的是以class作為索引類型的聯(lián)合數(shù)組。
module assoc_arr; class AB; int a; int b; endclass int arr[AB]; //Associative array 'arr' with class 'AB' as index AB obj, obj1; initial begin obj = new(); obj1= new(); arr[obj]=20; //Store 20 at the object handle index 'obj' $display("%0d",arr[obj]); arr[obj1]=10; //Store 10 at the object handle index 'obj1' $display("%0d",arr[obj1]); end endmodule
仿真log:
20 10 V C S S i m u l a t i o n R e p o r t
聲明類“AB”,并使用它作為關(guān)聯(lián)數(shù)組中的索引
int arr[AB]
聲明兩個AB類型的對象(obj和obj1),并實例化,賦值以這兩個對象為索引的聯(lián)合數(shù)組值。
arr[obj] = 20; arr[obj1] = 10;
String Index– Example
下面是一個以字符串為索引類型的聯(lián)合數(shù)組示例:
module assoc_arr; integer St [string] = '{"Peter":26, "Paul":24, "Mary":22}; integer data; initial begin $display("St=",St); $display("data stored at Peter = %0d",St["Peter"]); $display("data stored at Paul = %0d",St["Paul"]); $display("data stored at Mary = %0d",St["Mary"]); St["mike"] = 20; //new data stored at new index "mike" data = St["mike"]; //retrieve data stored at index "mike" $display("data stored at mike = %0d",data); $display("St=",St); end endmodule
仿真log:
run -all; # KERNEL: St='{"Mary":22, "Paul":24, "Peter":26} # KERNEL: data stored at Peter = 26 # KERNEL: data stored at Paul = 24 # KERNEL: data stored at Mary = 22 # KERNEL: data stored at mike = 20 # KERNEL: St='{"Mary":22, "Paul":24, "Peter":26, "mike":20} # KERNEL: Simulation has fnished.
聲明關(guān)聯(lián)數(shù)組“St”,并對其進(jìn)行初始化:
integer St [string] = '{"Peter":26, "Paul":24, "Mary":22};
意味著將26存儲在索引“Peter”中,24存儲在索引中
“Paul”,22存儲在索引“Mary”中。
2. 分別打印數(shù)組。
3. 在新索引“mike”中添加新數(shù)據(jù)。注意,這個數(shù)據(jù)項的內(nèi)存此時才開始分配。
Associative Array Methods
下面是關(guān)聯(lián)數(shù)組提供的一些方法。
module assoc_arr; int temp, imem[int]; integer St [string] = '{"Peter":20, "Paul":22, "Mary":23}; initial begin if(St.exists( "Peter") ) $display(" Index Peter exists "); //Assign data to imem[int] imem[ 2'd3 ] = 1; imem[ 16'hffff ] = 2; imem[ 4'b1000 ] = 3; $display( " imem has %0d entries", imem.num ); if(imem.exists( 4'b1000)) $display("Index 4b'1000 exist)"); imem.delete(4'b1000); if(imem.exists( 4'b1000)) $display("Index 4b'1000 exists)"); else $display(" Index 4b'1000 does not exist"); imem[ 4'b1000 ] = 3; if(imem.first(temp)) $display(" First entry is at index %0d ",temp); if(imem.next(temp)) $display(" Next entry is at index %0b ",temp); if(imem.last(temp)) $display(" Last entry is at index %0h",temp); imem.delete( ); //delete all entries $display(" imem has %0d entries", imem.num ); $display(" imem = %p", imem); end endmodule
仿真log:
Index Peter exists imem has 3 entries Index 4b'1000 exists Index 4b'1000 does not exist First entry is at index 3 Next entry is at index 1000 Last entry is at index ffff imem has 0 entries imem = '{} V C S Simulation Report
聲明了兩個關(guān)聯(lián)數(shù)組
int imem[int]; integer St [string] = '{"Peter":20, "Paul":22, "Mary":23};
使用.exists()判斷某個索引對應(yīng)的數(shù)據(jù)元素是否存在。
使用.num(),獲取該聯(lián)合數(shù)組具有的元素個數(shù)
使用.delete()刪除特定索引對應(yīng)的元素。如果不指定索引,則所有的元素都會被刪除。
使用方法first()、next()和last()訪問某個聯(lián)合數(shù)組元素
First entry is at index 3 Next entry is at index 1000 Last entry is at index ffff
Associative Array– Default Value
可以在關(guān)聯(lián)數(shù)組聲明時為其指定默認(rèn)值。
module assoc_arr; string words [int] = '{default: "hello"}; initial begin $display("words = %p", words['hffff]); //default $display("words = %p", words[0]); //default $display("words = %p", words[1000]); //default words['hffff] = "goodbye"; $display("words = %p", words); $display("words = %p", words[100]); //default end endmodule
仿真log:
words = "hello" words = "hello" words = "hello" words = '{0xffff:"goodbye"} words = "hello" V C S S i m u l a t i o n R e p o r t
數(shù)組“words”的初始化默認(rèn)值是“hello”,當(dāng)我們訪問索引“hffff”、“0”和“1000”時,都會打印默認(rèn)值“hello”。
我們?yōu)閕ndex ' hffff分配一個字符串值" goodbye "。
Creating aDynamic Array ofAssociative Arrays
關(guān)聯(lián)數(shù)組也可以是動態(tài)數(shù)組中的元素,如下示例:
module assoc_arr; //Create a dynamic array whose elements are associative arrays int games [ ] [string]; initial begin //Create a dynamic array with size of 3 elements games = new [3]; //Initialize the associative array inside each dynamic //array element games [0] = '{ "football" : 10,"baseball" : 20,"hututu":70 }; games [1] = '{ "cricket" : 30, "ice hockey" : 40 }; games [2] = '{ "soccer" : 50, "rugby" : 60 }; // Iterate through each element of dynamic array foreach (games[element]) // Iterate through each index of the current element in // dynamic array foreach (games[element][index]) $display ("games[%0d][%s] = %0d", element, index, games[element][index]); end endmodule
仿真log:
games[0][baseball] = 20 games[0][football] = 10 games[0][hututu] = 70 games[1][cricket] = 30 games[1][ice hockey] = 40 games[2][rugby] = 60 games[2][soccer] = 50 V C S S i m u l a t i o n R e p o r t
審核編輯:湯梓紅
-
Verilog
+關(guān)注
關(guān)注
28文章
1345瀏覽量
109986 -
System
+關(guān)注
關(guān)注
0文章
165瀏覽量
36885 -
數(shù)組
+關(guān)注
關(guān)注
1文章
416瀏覽量
25910
原文標(biāo)題:SystemVerilog中的關(guān)聯(lián)數(shù)組
文章出處:【微信號:芯片驗證工程師,微信公眾號:芯片驗證工程師】歡迎添加關(guān)注!文章轉(zhuǎn)載請注明出處。
發(fā)布評論請先 登錄
相關(guān)推薦
轉(zhuǎn)一篇Systemverilog的一個牛人總結(jié)
求職寶典:寒武紀(jì)2019筆試題
PHP數(shù)組排序
基于社交網(wǎng)絡(luò)和關(guān)聯(lián)數(shù)據(jù)的服務(wù)網(wǎng)絡(luò)構(gòu)建方法
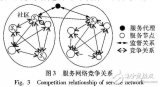
基于本體的軟件工程關(guān)聯(lián)數(shù)據(jù)的自動構(gòu)建
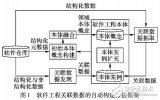
SystemVerilog中數(shù)組的賦值、索引和切片
SystemVerilog動態(tài)數(shù)組的大小更改展示
SystemVerilog中可以嵌套的數(shù)據(jù)結(jié)構(gòu)
網(wǎng)絡(luò)和變量的未壓縮數(shù)組
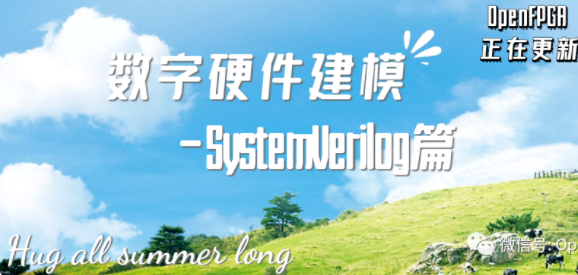
使用SystemVerilog解決數(shù)組問題
一些有趣的數(shù)組相關(guān)的SystemVerilog約束
一些有趣的數(shù)組相關(guān)的SystemVerilog約束
帶你了解SystemVerilog中的關(guān)聯(lián)數(shù)組
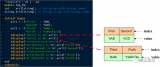
隨機(jī)抽取SV數(shù)組中的一個元素方法實現(xiàn)
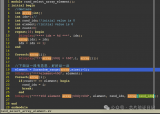
評論