前言
日常開發中,我們很多時候需要用到Java 8
的Lambda
表達式,它允許把函數作為一個方法的參數,讓我們的代碼更優雅、更簡潔。所以整理了一波工作中,我常用的,有哪些Lambda
表達式。看完一定會有幫助的。
基于 Spring Boot + MyBatis Plus + Vue & Element 實現的后臺管理系統 + 用戶小程序,支持 RBAC 動態權限、多租戶、數據權限、工作流、三方登錄、支付、短信、商城等功能
- 項目地址:https://github.com/YunaiV/ruoyi-vue-pro
- 視頻教程:https://doc.iocoder.cn/video/
1. list 轉 map
工作中,我們經常遇到list
轉map
的案例。Collectors.toMap
就可以把一個list
數組轉成一個Map
。代碼如下:
publicclassTestLambda{
publicstaticvoidmain(String[]args){
ListuserInfoList=newArrayList<>();
userInfoList.add(newUserInfo(1L,"芋道源碼",18));
userInfoList.add(newUserInfo(2L,"程序員芋艿",27));
userInfoList.add(newUserInfo(2L,"打代碼的芋艿",26));
/**
*list轉map
*使用Collectors.toMap的時候,如果有可以重復會報錯,所以需要加(k1,k2)->k1
*(k1,k2)->k1表示,如果有重復的key,則保留第一個,舍棄第二個
*/
MapuserInfoMap=userInfoList.stream().collect(Collectors.toMap(UserInfo::getUserId,userInfo->userInfo,(k1,k2)->k1));
userInfoMap.values().forEach(a->System.out.println(a.getUserName()));
}
}
//運行結果
芋道源碼
程序員芋艿
類似的,還有Collectors.toList()
、Collectors.toSet()
,表示把對應的流轉化為list
或者Set
。
基于 Spring Cloud Alibaba + Gateway + Nacos + RocketMQ + Vue & Element 實現的后臺管理系統 + 用戶小程序,支持 RBAC 動態權限、多租戶、數據權限、工作流、三方登錄、支付、短信、商城等功能
2. filter()過濾
從數組集合中,過濾掉不符合條件的元素,留下符合條件的元素。
ListuserInfoList=newArrayList<>();
userInfoList.add(newUserInfo(1L,"芋道源碼",18));
userInfoList.add(newUserInfo(2L,"程序員芋艿",27));
userInfoList.add(newUserInfo(3L,"打代碼的芋艿",26));
/**
*filter過濾,留下超過18歲的用戶
*/
ListuserInfoResultList=userInfoList.stream().filter(user->user.getAge()>18).collect(Collectors.toList());
userInfoResultList.forEach(a->System.out.println(a.getUserName()));
//運行結果
程序員芋艿
打代碼的芋艿
3. foreach 遍歷
foreach 遍歷list,遍歷map,真的很絲滑。
/**
*forEach遍歷集合List列表
*/
ListuserNameList=Arrays.asList("芋道源碼","程序員芋艿","艿艿");
userNameList.forEach(System.out::println);
HashMaphashMap=newHashMap<>();
hashMap.put("公眾號","芋道源碼");
hashMap.put("職業","程序員芋艿");
hashMap.put("昵稱","艿艿");
/**
*forEach遍歷集合Map
*/
hashMap.forEach((k,v)->System.out.println(k+": "+v));
//運行結果
芋道源碼
程序員芋艿
打代碼的芋艿
職業:程序員芋艿
公眾號:芋道源碼
昵稱:艿艿
4. groupingBy 分組
提到分組,相信大家都會想起SQL
的group by
。我們經常需要一個List做分組操作。比如,按城市分組用戶。在Java8之前,是這么實現的:
ListoriginUserInfoList=newArrayList<>();
originUserInfoList.add(newUserInfo(1L,"芋道源碼",18,"深圳"));
originUserInfoList.add(newUserInfo(3L,"打代碼的芋艿",26,"湛江"));
originUserInfoList.add(newUserInfo(2L,"程序員芋艿",27,"深圳"));
Map>result=newHashMap<>();
for(UserInfouserInfo:originUserInfoList){
Stringcity=userInfo.getCity();
ListuserInfos=result.get(city);
if(userInfos==null){
userInfos=newArrayList<>();
result.put(city,userInfos);
}
userInfos.add(userInfo);
}
而使用Java8的groupingBy
分組器,清爽無比:
Map>result=originUserInfoList.stream()
.collect(Collectors.groupingBy(UserInfo::getCity));
5. sorted+Comparator 排序
工作中,排序的需求比較多,使用sorted+Comparator
排序,真的很香。
ListuserInfoList=newArrayList<>();
userInfoList.add(newUserInfo(1L,"芋道源碼",18));
userInfoList.add(newUserInfo(3L,"打代碼的芋艿",26));
userInfoList.add(newUserInfo(2L,"程序員芋艿",27));
/**
*sorted+Comparator.comparing排序列表,
*/
userInfoList=userInfoList.stream().sorted(Comparator.comparing(UserInfo::getAge)).collect(Collectors.toList());
userInfoList.forEach(a->System.out.println(a.toString()));
System.out.println("開始降序排序");
/**
*如果想降序排序,則可以使用加reversed()
*/
userInfoList=userInfoList.stream().sorted(Comparator.comparing(UserInfo::getAge).reversed()).collect(Collectors.toList());
userInfoList.forEach(a->System.out.println(a.toString()));
//運行結果
UserInfo{userId=1,userName='芋道源碼',age=18}
UserInfo{userId=3,userName='打代碼的芋艿',age=26}
UserInfo{userId=2,userName='程序員芋艿',age=27}
開始降序排序
UserInfo{userId=2,userName='程序員芋艿',age=27}
UserInfo{userId=3,userName='打代碼的芋艿',age=26}
UserInfo{userId=1,userName='芋道源碼',age=18}
6.distinct 去重
distinct
可以去除重復的元素:
Listlist=Arrays.asList("A","B","F","A","C");
Listtemp=list.stream().distinct().collect(Collectors.toList());
temp.forEach(System.out::println);
7. findFirst 返回第一個
findFirst
很多業務場景,我們只需要返回集合的第一個元素即可:
Listlist=Arrays.asList("A","B","F","A","C");
list.stream().findFirst().ifPresent(System.out::println);
8. anyMatch 是否至少匹配一個元素
anyMatch
檢查流是否包含至少一個滿足給定謂詞的元素。
Streamstream=Stream.of("A","B","C","D");
booleanmatch=stream.anyMatch(s->s.contains("C"));
System.out.println(match);
//輸出
true
9. allMatch 匹配所有元素
allMatch
檢查流是否所有都滿足給定謂詞的元素。
Streamstream=Stream.of("A","B","C","D");
booleanmatch=stream.allMatch(s->s.contains("C"));
System.out.println(match);
//輸出
false
10. map 轉換
map
方法可以幫我們做元素轉換,比如一個元素所有字母轉化為大寫,又或者把獲取一個元素對象的某個屬性,demo
如下:
Listlist=Arrays.asList("jay","tianluo");
//轉化為大寫
ListupperCaselist=list.stream().map(String::toUpperCase).collect(Collectors.toList());
upperCaselist.forEach(System.out::println);
11. Reduce
Reduce可以合并流的元素,并生成一個值
intsum=Stream.of(1,2,3,4).reduce(0,(a,b)->a+b);
System.out.println(sum);
12. peek 打印個日志
peek()
方法是一個中間Stream
操作,有時候我們可以使用peek
來打印日志。
Listresult=Stream.of("程序員芋艿","芋道源碼","打代碼的芋艿")
.filter(a->a.contains("芋艿"))
.peek(a->System.out.println("關注公眾號:"+a)).collect(Collectors.toList());
System.out.println(result);
//運行結果
關注公眾號:程序員芋艿
關注公眾號:芋道源碼
[程序員芋艿,芋道源碼]
13. Max,Min 最大最小
使用lambda流求最大,最小值,非常方便。
ListuserInfoList=newArrayList<>();
userInfoList.add(newUserInfo(1L,"芋道源碼",18));
userInfoList.add(newUserInfo(3L,"打代碼的芋艿",26));
userInfoList.add(newUserInfo(2L,"程序員芋艿",27));
OptionalmaxAgeUserInfoOpt=userInfoList.stream().max(Comparator.comparing(UserInfo::getAge));
maxAgeUserInfoOpt.ifPresent(userInfo->System.out.println("maxageuser:"+userInfo));
OptionalminAgeUserInfoOpt=userInfoList.stream().min(Comparator.comparing(UserInfo::getAge));
minAgeUserInfoOpt.ifPresent(userInfo->System.out.println("minageuser:"+userInfo));
//運行結果
maxageuser:UserInfo{userId=2,userName='程序員芋艿',age=27}
minageuser:UserInfo{userId=1,userName='芋道源碼',age=18}
14. count 統計
一般count()
表示獲取流數據元素總數。
ListuserInfoList=newArrayList<>();
userInfoList.add(newUserInfo(1L,"芋道源碼",18));
userInfoList.add(newUserInfo(3L,"打代碼的芋艿",26));
userInfoList.add(newUserInfo(2L,"程序員芋艿",27));
longcount=userInfoList.stream().filter(user->user.getAge()>18).count();
System.out.println("大于18歲的用戶:"+count);
//輸出
大于18歲的用戶:2
15. 常用函數式接口
其實lambda離不開函數式接口,我們來看下JDK8常用的幾個函數式接口:
-
Function
(轉換型): 接受一個輸入參數,返回一個結果 -
Consumer
(消費型): 接收一個輸入參數,并且無返回操作 -
Predicate
(判斷型): 接收一個輸入參數,并且返回布爾值結果 -
Supplier
(供給型): 無參數,返回結果
Function
是一個功能轉換型的接口,可以把將一種類型的數據轉化為另外一種類型的數據
privatevoidtestFunction(){
//獲取每個字符串的長度,并且返回
Functionfunction=String::length;
Streamstream=Stream.of("程序員芋艿","芋道源碼","打代碼的芋艿");
StreamresultStream=stream.map(function);
resultStream.forEach(System.out::println);
}
Consumer
是一個消費性接口,通過傳入參數,并且無返回的操作
privatevoidtestComsumer(){
//獲取每個字符串的長度,并且返回
Consumercomsumer=System.out::println;
Streamstream=Stream.of("程序員芋艿","芋道源碼","打代碼的芋艿");
stream.forEach(comsumer);
}
Predicate
是一個判斷型接口,并且返回布爾值結果.
privatevoidtestPredicate(){
//獲取每個字符串的長度,并且返回
Predicatepredicate=a->a>18;
UserInfouserInfo=newUserInfo(2L,"程序員芋艿",27);
System.out.println(predicate.test(userInfo.getAge()));
}
Supplier
是一個供給型接口,無參數,有返回結果。
privatevoidtestSupplier(){
Suppliersupplier=()->Integer.valueOf("666");
System.out.println(supplier.get());
}
這幾個函數在日常開發中,也是可以靈活應用的,比如我們DAO操作完數據庫,是會有個result的整型結果返回。我們就可以用Supplier
來統一判斷是否操作成功。如下:
privatevoidsaveDb(Suppliersupplier) {
if(supplier.get()>0){
System.out.println("插入數據庫成功");
}else{
System.out.println("插入數據庫失敗");
}
}
@Test
publicvoidadd()throwsException{
Coursecourse=newCourse();
course.setCname("java");
course.setUserId(100L);
course.setCstatus("Normal");
saveDb(()->courseMapper.insert(course));
}
審核編輯 :李倩
-
JAVA
+關注
關注
19文章
2960瀏覽量
104565 -
代碼
+關注
關注
30文章
4753瀏覽量
68368 -
Lambda
+關注
關注
0文章
28瀏覽量
9859
原文標題:我用Lambda表達式寫代碼,開發速度提高了10倍!
文章出處:【微信號:芋道源碼,微信公眾號:芋道源碼】歡迎添加關注!文章轉載請注明出處。
發布評論請先 登錄
相關推薦
Verilog表達式的位寬確定規則
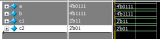
TestStand表達式中常用的語法規則和運算符使用
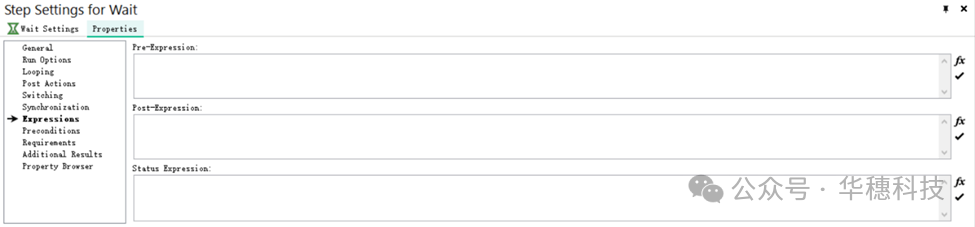
鴻蒙原生應用元服務開發-倉頡基本概念表達式(二)
鴻蒙原生應用元服務開發-倉頡基本概念表達式(一)
求助,有關表達式選項卡(ADS)的問題求解
mapgis屬性篩選表達式
西門子博途的算術表達式
rs觸發器的邏輯表達式
全加器的邏輯表達式怎么推
GVIM正則表達式介紹和使用示例

一文詳解Java表達式引擎選型
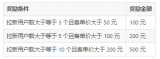
評論