在用到linux編程的時候,Makefile可以很好的幫助管理C語言工程,如何構建一個靜態(tài)庫,用一個很小的案例來說明。
首先準備需要的文件,以及文件中的內容,如下所示
$ cat test1.c
#include
int main()
{
printf("hello world\\n");
return 0;
}
這個.c文件非常簡單,就是輸出一個hello world。用gcc編譯,就能輸出。
`
: ~/Documents/clan/test1$ gcc test1.c
:~ /Documents/clan/test1$ tree
.
├── a.out
└── test1.c
0 directories, 3 files
:~/Documents/clan/test1$ ./a.out
hello world
現在換種方式實現,采用Makefile的形式,編輯Makefile的腳本
:~/Documents/clan/test1$ rm a.out
:~/Documents/clan/test1$ cat Makefile
test1 : test1.o
gcc -o test1 test1.o
test1.o : test1.c
gcc -c -o test1.o test1.c
clean :
rm -f test1 test1.o
編譯Makefile文件,同樣可以實現這個效果
:~/Documents/clan/test1$ tree
.
├── Makefile
└── test1.c
0 directories, 2 files
:/Documents/clan/test1$ make/Documents/clan/test1$ tree
gcc -c -o test1.o test1.c
gcc -o test1 test1.o
:
.
├── Makefile
├── test1
├── test1.c
└── test1.o
0 directories, 4 files
:~/Documents/clan/test1$ ./test1
hello world
現在將產物刪掉,方便后面的實驗
: ~/Documents/clan/test1$ make clean
rm -f test1 test1.o
:~ /Documents/clan/test1$ tree
.
├── Makefile
└── test1.c
0 directories, 2 files
現在回到gcc 編譯的過程中,先編譯得到.o文件,然后編譯得到靜態(tài)庫文件,最后通過編譯庫文件,同樣可以生成可執(zhí)行文件
: ~/Documents/clan/test1$ gcc -c -o test1.o test1.c
:~ /Documents/clan/test1$ tree
.
├── Makefile
├── test1.c
└── test1.o
0 directories, 3 files
: ~/Documents/clan/test1$ ar -cr libtest1.a test1.o
:~ /Documents/clan/test1$ tree
.
├── libtest1.a
├── Makefile
├── test1.c
└── test1.o
0 directories, 4 files
: ~/Documents/clan/test1$ gcc libtest1.a
:~ /Documents/clan/test1$ tree
.
├── a.out
├── libtest1.a
├── Makefile
├── test1.c
└── test1.o
0 directories, 5 files
:~/Documents/clan/test1$ ./a.out
hello world
刪除上述生成的文件
: ~/Documents/clan/test1$ rm a.out
:~ /Documents/clan/test1$ rm libtest1.a
: ~/Documents/clan/test1$ rm test1.o
:~ /Documents/clan/test1$ tree
.
├── Makefile
└── test1.c
0 directories, 2 files
重新編輯Makefile文件
test1 : libtest1.a
gcc -o test1 libtest1.a
libtest1.a : test1.o
ar -cr libtest1.a test1.o
test1.o : test1.c
gcc -c -o test1.o test1.c
clean :
rm -f test1 test1.o libtest1.a
重新編譯Makefile文件,然后執(zhí)行,同樣可以實現,這就是靜態(tài)庫的實現方式
: ~/Documents/clan/test1$ make
gcc -c -o test1.o test1.c
ar -cr libtest1.a test1.o
gcc -o test1 libtest1.a
:~ /Documents/clan/test1$ tree
.
├── libtest1.a
├── Makefile
├── test1
├── test1.c
└── test1.o
0 directories, 5 files
:~/Documents/clan/test1$ ./test1
hello world
上述方式,實現了一個非常簡單的案例,這是在同一目錄層級下實現的,如果涉及到多個目錄層級呢?
├── func
│ ├── func.c
│ └── func.h
├── Makefile
└── test1.c
其中func.c文件的代碼如下
#include "func.h"
int add(int a, int b){return a+b;}
func.h文件的代碼
int add(int a, int b);
test.c文件的代碼
#include
#include "func/func.h"
int main()
{
printf("hello world\\n");
printf("%d\\n",add(10,20));
return 0;
}
用gcc命令編譯
然后修改Makefile文件
:~/Documents/clan/test1$ cat Makefile
test1 : test1.o func/func.o
gcc -o test1 test1.o func/func.o
test1.o : test1.c
gcc -c -o test1.o test1.c
func/func.o : func/func.c func/func.h
gcc -c -o func/func.o func/func.c
clean :
rm -f test1 test1.o func/func.o
編譯所有文件,運行可執(zhí)行文件,即可輸出結果
:~/Documents/clan/test1$ tree
.
├── func
│ ├── func.c
│ └── func.h
├── Makefile
└── test1.c
1 directory, 4 files
: ~/Documents/clan/test1$ make
gcc -c -o test1.o test1.c
gcc -c -o func/func.o func/func.c
gcc -o test1 test1.o func/func.o
:~ /Documents/clan/test1$ tree
.
├── func
│ ├── func.c
│ ├── func.h
│ └── func.o
├── Makefile
├── test1
├── test1.c
└── test1.o
1 directory, 7 files
:~/Documents/clan/test1$ ./test1
hello world
30
要生成Makefile的靜態(tài)庫,只需要在這個基礎上進行修改即可
test1 : libtest1.a func/libfunc.a
gcc -o test1 libtest1.a func/libfunc.a
libtest1.a : test1.o
ar -cr libtest1.a test1.o
func/libfunc.a : func/func.o
ar -cr func/libfunc.a func/func.o
test1.o : test1.c
gcc -c -o test1.o test1.c
func/func.o : func/func.c func/func.h
gcc -c -o func/func.o func/func.c
clean :
rm -f test1 test1.o libtest1.a func/libfunc.a func/func.o
這是一個非常簡單的模板案例,靜態(tài)庫的編譯過程比較簡單,動態(tài)庫相對復雜。
審核編輯:劉清
-
C語言
+關注
關注
180文章
7598瀏覽量
136210 -
gcc編譯器
+關注
關注
0文章
78瀏覽量
3363 -
Linux編程
+關注
關注
0文章
5瀏覽量
614
發(fā)布評論請先 登錄
相關推薦
C語言標準庫的基本使用
靜態(tài)庫的優(yōu)點及其靜態(tài)庫的使用解析
如何利用C語言去調用rust靜態(tài)庫呢
學習C語言的目標和方法有哪些及C語言的關鍵字說明
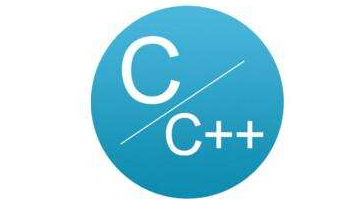
C語言宏定義與預處理、函數和函數庫
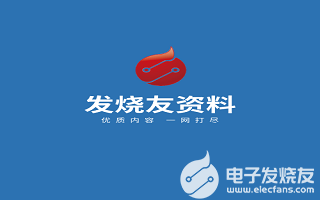
靜態(tài)庫和動態(tài)庫的生成以及使用(樹莓派)
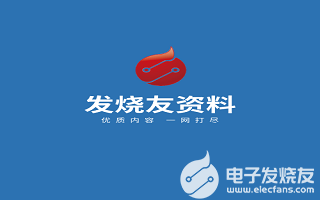
評論