代碼地址:https://github.com/Snowstorm0/learn-async
1 線程同步和異步
線程同步 :A線程要請求某個資源,但是此資源正在被B線程使用中,因為同步機制存在,A只能等待下去。耗時較長,安全性較高。
線程異步 :A線程要請求某個資源,但是此資源正在被B線程使用中,因為沒有同步機制存在,A線程仍然請求的到。
一個進程啟動的多個不相干的進程,他們之間的相互關系為異步;同步必須執行到底后才能執行其他操作,異步可同時執行。
多個線程執行的時候需要同步,如果是單線程則不需要同步。
2 異步實例
主方法和被調用的方法必須是不同的類,才能實現多線程。
2.1 啟動類
使用@EnableAsync
來開啟 SpringBoot 對于異步任務的支持。
Application:
@SpringBootApplication
@EnableAsync
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
2.2 線程池
配置類實現接口AsyncConfigurator,返回一個ThreadPoolTaskExecutor線程池對象。
config/AsyncConfig:
@Configuration
@EnableAsync
public class AsyncConfig implements AsyncConfigurer {
// ThredPoolTaskExcutor的處理流程
// 當池子大小小于corePoolSize,就新建線程,并處理請求
// 當池子大小等于corePoolSize,把請求放入workQueue中,池子里的空閑線程就去workQueue中取任務并處理
// 當workQueue放不下任務時,就新建線程入池,并處理請求,如果池子大小撐到了maximumPoolSize,就用RejectedExecutionHandler來做拒絕處理
// 當池子的線程數大于corePoolSize時,多余的線程會等待keepAliveTime長時間,如果無請求可處理就自行銷毀
@Override
@Bean
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
// 核心線程數:線程池創建的時候初始化的線程數
executor.setCorePoolSize(10);
// 最大線程數:線程池最大的線程數,只有緩沖隊列滿了之后才會申請超過核心線程數的線程
executor.setMaxPoolSize(100);
// 緩沖隊列:用來緩沖執行任務的隊列
executor.setQueueCapacity(50);
// 線程池關閉:等待所有任務都完成再關閉
executor.setWaitForTasksToCompleteOnShutdown(true);
// 等待時間:等待5秒后強制停止
executor.setAwaitTerminationSeconds(5);
// 允許空閑時間:超過核心線程之外的線程到達60秒后會被銷毀
executor.setKeepAliveSeconds(60);
// 線程名稱前綴
executor.setThreadNamePrefix("learn-Async-");
// 初始化線程
executor.initialize();
return executor;
}
@Override
public AsyncUncaughtExceptionHandler getAsyncUncaughtExceptionHandler() {
return null;
}
}
2.3 controller
通過該層調用測試 Async。
@RestController
@RequestMapping("/homepage")
public class AsyncController {
@Autowired
AsyncService asyncTaskService;
@GetMapping("/learnAsync")
public String learnAsync(){
for (int i = 0; i < 10; i++) {
asyncTaskService.executeAsyncTask(i);
}
return "1";
}
}
2.4 service
通過@Async
注解表明該方法是異步方法,如果注解在類上,那表明這個類里面的所有方法都是異步的。
@Service
public class AsyncService {
private final static Logger logger = LoggerFactory.getLogger(com.spring.boot.service.AsyncService.class);
@Async // 表明該方法是異步方法。如果注解在類上,那表明類里面的所有方法都是異步
public void executeAsyncTask(int i) {
logger.info("\\t 完成任務" + i);
System.out.println("線程" + Thread.currentThread().getName() + " 執行異步任務:" + i);
}
}
2.5 輸出
3 Future 類
修改service層,分別使用同步調用、異步調用無返回、異步調用使用 Future 返回。
3.1 同步調用
public long subBySync() throws Exception {
long start = System.currentTimeMillis();
long sum = 0;
long end = System.currentTimeMillis();
sum = end - start;
return sum;
}
3.2 異步調用無返回
@Async
public void subByVoid() throws Exception {
long start = System.currentTimeMillis();
long sum = 0;
long end = System.currentTimeMillis();
sum = end - start;
}
3.3 異步調用 Future 返回
controller:
Future task = asyncTaskService.subByAsync();
service:
@Async
public Future subByAsync() throws Exception {
long start = System.currentTimeMillis();
long sum = 0;
long end = System.currentTimeMillis();
sum = end - start;
return new AsyncResult<>(sum);
}
4 CompletableFuture 類
若使用 Future 出現報錯:
無法判斷org.springframework.scheduling.annotation.AsyncResult<>的類型參數
不存在類型變量V的實例,使org.springframework.scheduling.annotation.AsyncResult符合XXX
可以使用 CompletableFuture 類:
@Asyncpublic
CompletableFuture
5 線程關閉
當線程數量超過核心線程數量之后,運行完畢的舊的線程會被關閉。
可以通過定時任務測試。
batch/ScheduledTaskService:
@Component
@EnableScheduling
public class ScheduledTaskService {
@Autowired
AsyncService asyncService;
@Scheduled(cron = "1/1 * * * * ? ") //1s一次
public void learnCron(){
asyncService.learnScheduledAsync();
}
}
在 AsyncService 添加方法:
// 使用定時任務調用此方法創建線程
@Async
public void learnScheduledAsync(){
Long timeLong = System.currentTimeMillis();
SimpleDateFormat timeFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); //設置格式
String timeString = timeFormat.format(timeLong);
System.out.println("線程" + Thread.currentThread().getName());
System.out.println("timeString:" + timeString + "\\n");
}
在異步配置(AsyncConfig)中已設置核心線程數為10:
// 核心線程數:線程池創建的時候初始化的線程數
executor.setCorePoolSize(10);
運行可以觀察輸出,線程數達到10后會再一次從1開始。
審核編輯:湯梓紅
-
JAVA
+關注
關注
19文章
2942瀏覽量
104081 -
多線程
+關注
關注
0文章
275瀏覽量
19848 -
spring
+關注
關注
0文章
335瀏覽量
14254 -
Boot
+關注
關注
0文章
148瀏覽量
35672 -
SpringBoot
+關注
關注
0文章
172瀏覽量
145
發布評論請先 登錄
相關推薦
Java多線程的用法
利用線程的互斥實現串口多線程收發數據
多線程與聊天室程序的創建
多線程好還是單線程好?單線程和多線程的區別 優缺點分析
mfc多線程編程實例及代碼,mfc多線程間通信介紹
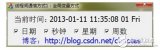
評論