介紹
本示例使用[@ohos.WorkSchedulerExtensionAbility] 、[@ohos.net.http]、[@ohos.notification] 、[@ohos.bundle]、[@ohos.fileio] 等接口,實現了設置后臺任務、下載更新包 、保存更新包、發送通知 、安裝更新包實現升級的功能。
效果預覽
使用說明
- 安裝本應用之前,先編譯好未簽名的應用包,然后在終端執行工程里的腳本,目錄為:WorkScheduler/signTool/b_sign_hap_release.bat;
- 未連接wifi狀態下進入應用;
- 進入首頁后連接wifi;
- 后臺判斷版本號后會下載新的升級包,并在頁面中給出彈窗詢問是否安裝,點擊“確定”按鈕;
- 應用會安裝已經下載的升級包,實現版本更新,安裝后會回到設備桌面,此時點擊應用圖標,可以看到版本已經是新版本了。
- 運行自動化測試用例時,必須使用命令行裝包,不能使用ide自動裝包,安裝自動化測試包之前,先編譯好未簽名的測試包, 然后在終端執行工程里的腳本,目錄為:WorkScheduler/signTool/a_sign_hap_release.bat;
- 運行自動化測試應用時需要使用如下命令:
hdc shell aa test -b ohos.samples.workschedulerextensionability -m entry_test -s unittest OpenHarmonyTestRunner -s class ActsAbilityTest -s timeout 150000
代碼解讀
entry/src/main/ets/
|---Application
| |---MyAbilityStage.ets // 入口文件
|---feature
| |---WorkSchedulerSystem.ets // 封裝各個功能接口
|---MainAbility
| |---MainAbility.ets // 請求權限
|---pages
| |---Index.ets // 首頁
|---util
| |---Logger.ets // 日志文件
|---WorkSchedulerAbility
| |---WorkSchedulerAbility.ets // 延時任務觸發后的回調
鴻蒙HarmonyOS與OpenHarmony技術
+mau123789是v直接拿取
具體實現
鴻蒙next開發知識更新在:[gitee.com/li-shizhen-skin/harmony-os/blob/master/README.md
]
- 設置延時任務、下載更新包、保存更新包、發送通知、安裝更新包的功能接口都封裝在WorkSchedulerSystem中, 源碼參考:[WorkSchedulerSystem.ets]
/*
* Copyright (c) 2023-2024 Huawei Device Co., Ltd.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import fs from '@ohos.file.fs';
import notificationManager from '@ohos.notificationManager';
import Notification from '@ohos.notification';
import bundle from '@ohos.bundle.installer';
import account from '@ohos.account.osAccount';
import workScheduler from '@ohos.resourceschedule.workScheduler';
import http from '@ohos.net.http';
import { Logger } from '../utils/Logger';
const FILE_NAME = '/UpdateWorkScheduler.hap';
const BUNDLE_NAMES = ['ohos.samples.workschedulerextensionability'];
const INSTALL_PARAMETER = 1;
export namespace WorkSchedulerSystem {
/**
* Store the file to the specified directory.
*
* @param pathDir Path to save the file.
* @param content The contents of the file to be saved.
*/
export function saveFile(pathDir: string, content: ArrayBuffer): void {
try {
let filePath = pathDir + FILE_NAME;
let fd = fs.openSync(filePath, 0o2 | 0o100).fd;
fs.writeSync(fd, content);
fs.closeSync(fd);
} catch (err) {
Logger.error(`saveFile failed, code is ${err.code}, message is ${err.message}`);
}
}
/**
* Sending a Notification.
*
* @param bundleName Check the name of the application that has permission.
* @permission ohos.permission.NOTIFICATION_CONTROLLER
*/
export async function handleNotification(bundleName: string): Promise< void > {
await notificationManager.requestEnableNotification();
Notification.subscribe({
onConsume: (data) = > {
if (data.request.content.normal.text === 'isReady') {
AppStorage.SetOrCreate('isShowDialog', true);
}
}
}, {
bundleNames: BUNDLE_NAMES
})
}
/**
* Publishes a notification of the specified content.
*
* @param title Title of Notice.
* @param text Content of Notification Text.
* @param additionalText Additional text.
* @permission ohos.permission.NOTIFICATION_CONTROLLER
*/
export function publishNotification(title: string, text: string, additionalText: string): void {
notificationManager.publish({
content: {
contentType: Notification.ContentType.NOTIFICATION_CONTENT_BASIC_TEXT,
normal: {
title,
text,
additionalText
}
}
})
}
/**
* Install the application package in the specified path.
*
* @param filePath An array of paths to hold the installation package.
* @permission ohos.permission.INSTALL_BUNDLE
*/
export async function installBundle(filePath: Array< string >): Promise< void > {
try {
let bundleInstall = await bundle.getBundleInstaller();
let userId = await account.getAccountManager().getOsAccountLocalIdFromProcess();
bundleInstall.install(filePath, {
userId: userId,
installFlag: INSTALL_PARAMETER,
isKeepData: false
}, (status, statusMessage) = > {
Logger.info(`installBundle filepath is ${filePath}`);
Logger.info(`installBundle code is ${status.code}, message is ${JSON.stringify(statusMessage)}`);
})
} catch (err) {
Logger.error(`installBundle failed, code is ${err.code}, message is ${err.message}`);
}
}
/**
* Register the delayed task and pass the parameters.
*
* @param version Current application version.
* @param bundleName The name of the application package for which the task needs to be registered.
* @param filePath Storage address of the application package.
*/
export async function startUpdateSample(version: string, bundleName: string, filePath: string): Promise< void > {
try {
let workInfo = {
workId: 1,
bundleName: bundleName,
abilityName: 'WorkSchedulerAbility',
networkType: workScheduler.NetworkType.NETWORK_TYPE_WIFI,
parameters: {
version: version,
filePath: filePath
}
};
workScheduler.startWork(workInfo);
}
catch (err) {
Logger.error(`startWork failed, code is ${err.code}, message is ${err.message}`);
}
}
/**
* Register the delayed task and pass the parameters.
*
* @param url Url of the application package.
* @permission ohos.permission.INTERNET
*/
export async function getNewHap(url: string): Promise< http.HttpResponse > {
try {
return await http.createHttp().request(
url,
{
expectDataType: http.HttpDataType.ARRAY_BUFFER
});
} catch (err) {
Logger.error(`get result failed, code is ${err.code}, message is ${err.message}`);
}
}
}
- 設置延時任務:在運行示例時會在[MainAbility.ets] 通過WorkSchedulerSystem.startUpdateSample()方法調用workScheduler.startWork()建立任務;
- 下載更新包:當任務條件滿足后,會在[WorkSchedulerAbility.ets]通過WorkSchedulerSystem.getNewHap()方法調用http.createHttp().request()接口下載需要的文件;
- 保存更新包:通過WorkSchedulerSystem.saveFile()來實現,受限調用fileio.openSync()創建文件,然后調用fileio.writeSync()將下載的內容寫入指定文件內;
- 發送通知:在[WorkSchedulerAbility.ets] 中通過WorkSchedulerSystem.publishNotification()方法,調用Notification.publish()接口發送指定內容的信息;
- 接收通知:在[MainAbility.ets]中通過WorkSchedulerSystem.handleNotification()方法調用Notification.subscribe()接口獲取信息,根據信息內容決定是否提示用戶升級;
- 安裝更新包:在[WorkSchedulerAbility.ets] 通過WorkSchedulerSystem.installBundle()方法實現,首先調用bundle.getBundleInstaller()獲取Installer對象,然后調用bundleInstall.install()接口實現裝包,完成升級。
審核編輯 黃宇
聲明:本文內容及配圖由入駐作者撰寫或者入駐合作網站授權轉載。文章觀點僅代表作者本人,不代表電子發燒友網立場。文章及其配圖僅供工程師學習之用,如有內容侵權或者其他違規問題,請聯系本站處理。
舉報投訴
-
WIFI
+關注
關注
81文章
5255瀏覽量
201684 -
鴻蒙
+關注
關注
56文章
2267瀏覽量
42480 -
HarmonyOS
+關注
關注
79文章
1946瀏覽量
29732 -
OpenHarmony
+關注
關注
25文章
3545瀏覽量
15731
發布評論請先 登錄
相關推薦
UCOSiii任務延時時間達到問題
之前一個帖子發錯版塊了當延時時間到了后,時鐘節拍任務會把該等待延時的任務放入任務就緒表中,在尋找最高優先級的函數中會找到優先級最高的就緒
發表于 04-06 04:36
UCOSIII延時函數任務怎么調度?
OSTimeDlyHMSM(0,0,0,10,OS_OPT_TIME_PERIODIC,&err);延時10ms。對于這樣的延時函數,會觸發任務調度。我的問題是
發表于 04-10 04:36
UCOSIII任務中使用延時函數進行調度怎么設置?
請教各位大神關于UCOSIII任務中使用延時函數進行調度的問題:假如我有4個串口中斷,都是每隔500ms使用任務內建消息隊列的OSTaskQPost向處理
發表于 05-11 03:07
FreeRTOS如何使用delay作為系統延時、任務調度
請教一個問題,最近在學習使用FreeRTOS,想像原子一樣在delay.c里添加RTOS的系統支持,即使用tick時鐘作延時?,F在有幾個問題:1、在啟動任務調度器前,如果調用了delay_ms
發表于 06-10 04:37
HarmonyOS應用開發-分布式任務調度
,體驗HarmonyOS的分布式任務調度。您將建立什么在這個CodeLab中,你將創建DemoProject,并將Demo編譯成HAP,此示例應用程序展示了如何使用分布式任務
發表于 09-18 09:21
最遲預分配容錯實時調度算法設計與分析
提出一種多類型任務集的容錯實時調度算法,詳細分析該算法的調度機制,證明了該算法的正確性,并給出了該算法的可調度條件,最后通過模擬實驗分析了算法的性能。實驗表
發表于 11-20 12:01
?17次下載
分時調度思想在單片機應用中的一個實例
利用分時操作系統中的分時調度思想可以使一個多終端的系統快速響應各終端的要求。本文首先介紹分時操作系統中的分時調度思想, 然后以程控交換機的控制系統為例, 在簡介控
發表于 07-17 16:03
?26次下載
云任務閾值調度算法
針對當前云任務調度算法在密碼云環境中無法實現任務實時處理的問題,提出一種基于滾動優化窗口的實時閾值調度方法。首先,將密鑰調用環節融入密碼任務
發表于 11-24 17:08
?5次下載
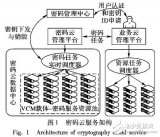
移動終端最優節能任務調度
約束條件下調度的規律性,提出按最近截止時間進行分組調度算法,每組調度采用動態最優化策略進行決策。實驗結果表明,該算法在任務可調度的情況下能夠
發表于 02-07 16:30
?1次下載
鴻蒙OS 分布式任務調度
鴻蒙OS 分布式任務調度概述 在 HarmonyO S中,分布式任務調度平臺對搭載 HarmonyOS 的多設備構筑的“超級虛擬終端”提供統
評論